HTML
HTML-Fundamentals
Target
The target attribute tells the <a>
element where to open the link. For instance, _blank
will tell the browser to open the link in a new tab.
<div>
<p id="my-example">
This is my example paragraph.</p>
</div>
<a href="#my-example">
Show me my example please.</a>
HTML-Semantics
Semantic HTML is simply writing HTML with further specification of the documents sections, such as using
elements that give more meaning and detail to your document, some of which will be listed below.
<article>
<aside>
<details>
<figcaption>
<figure>
<footer>
<header>
<main>
<mark>
<nav>
<section>
<summary>
<time>
HTML-Tables
Table Elements
<table> Element |
<thead> Element |
<tr> Element |
<td> Element |
<tbody> Element |
<tfooter> Element |
---|---|---|---|---|---|
<table> element is used to display a table in HTML. |
<thead> element is used within the table sections which you specify to be the head sections. |
<tr> is used to add more rows to the HTML-table. |
<td> element is used to add cells of data to your table, nested within a row element. |
<tbody> element is semantic and will contain all table data. |
<tfooter> Specifies the bottom section of a table element. |
Table Element Attributes
Colspan | Rolspan |
---|---|
Colspan value default is 1 , and can go as high as 1000 . |
Rowspan value default is 1 , and can go as high as65534 . |
States how many columns the cell should span.(Vertical) | States how many rows the cell should span.(Horizontal) |
CSS
Class Selectors | ID Selectors |
---|---|
Can be reusable | Should be unique |
Can be applied to many elements | Should be used to style only a single element |
Uses a . to select class-name |
Uses a # to select the id-name |
Z-Index
z-index
is a CSS property, specifying depth of an elements apperance in the possible scenario of it overlapping other elements.
It holds a default value of auto
.
z-index
can be whole number values(1, 100, -1, etc)
, if not the default value, and basically, elements holding a higher z-index
value will be on top of the lower value elements.
Property: Display
display
is the specification of which type of rendering box for an element.
Some more commonly used values
are;
inline-block
elements- Can be side-by-side.
- Allows for adjustments for the height and width done manually.
block
elements- Will take the full width of parent elements
- Before and after line breaks
- Also allows adjustments for the height and width manually
inline
elements- Least amount of space as possible is taken up.
- Horizontally flowing
- Does not allow for adjustments for width and height.
CSS Positions
The CSS position
property can hold 1 of 4 values which are the following possiblities:
Fixed
- It will be fixed to a specified location on the page
- Good for making an element accessible to the user at all times no matter where they scroll to on page.
Static
- Default value of the
position
property. - No effect on the positioning of an element.
Relative
- Allows element position to be specified relative to original location on page.
- Determination of the actual position of element, relative to its original can be done with offset properties.
- Positioning will act as default,
static
value if there is no offset properties used.
Absolute
absolute
value removes element from the flow of the document, allowing overlapping of elements.- In relation to the elements closest parent, is where the element in discussion is placed. (Relative to nearest parent element).
- Additionally, if the element has no parents, it will simply use the
<body>
of the document, moving along the page with scrolling.
Float
Within the parent element, float
specifies horizontally where an element should be. Or, where it should float
inside the parent element.
Float: Values
- Left:Element will
float
to the left of it's container. - Right:Element will
float
to the right of it's container.
width
of container needs specification, otherwise element will determine the value as the full width of parent element.
Clear
When two elements whom share a parent element collide with one another, the clear
property is what can be used to determine the behavior of an element.
More commonly used along with the previously discussed, float
property. Allows for specification on the desired side a floating element should float
to.
CSS-Box-Model
The CSS Box model comprise a set of properties which define parts of an element that take space on a webpage.
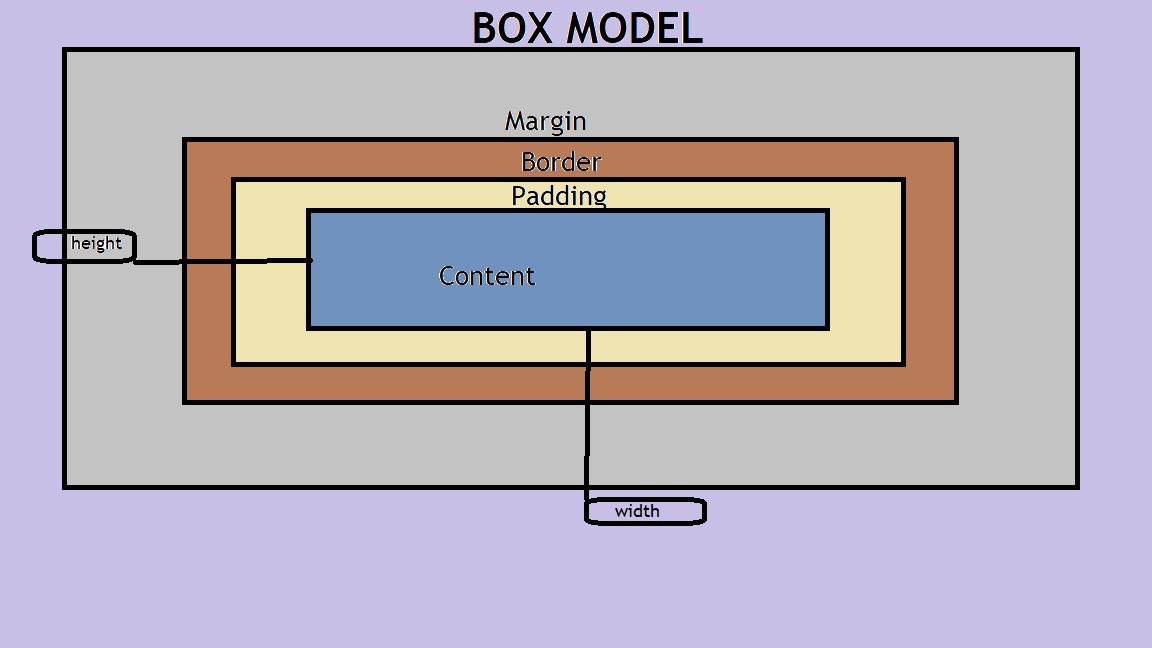
Box Model includes:
- Content area's size(width and height)
- The following elements;
- Padding- Amount of space between content area and border.
- Border- Thickness & style of border surrounding content area & padding.
- Margin-Amount of space between border and outside edge of an element.
Box-Model: Box-Sizing
As previously discussed, the box which is wrapped around an HTML element is the CSS:Box-Model.
CSS:Box-Model has a property in which the height and width properties are controlled, which is known as the box-sizing
property.
Default Value: is set to content-box
. Actual size of element is rendered, including content-box. (No padding or borders
Another value: is border-box
which allows padding and border of an element to be included in the total width and height.(This value is widely preferred by most devs, in lue of the previously discussed valuecontent-box
)
Box-Model:Content-Box
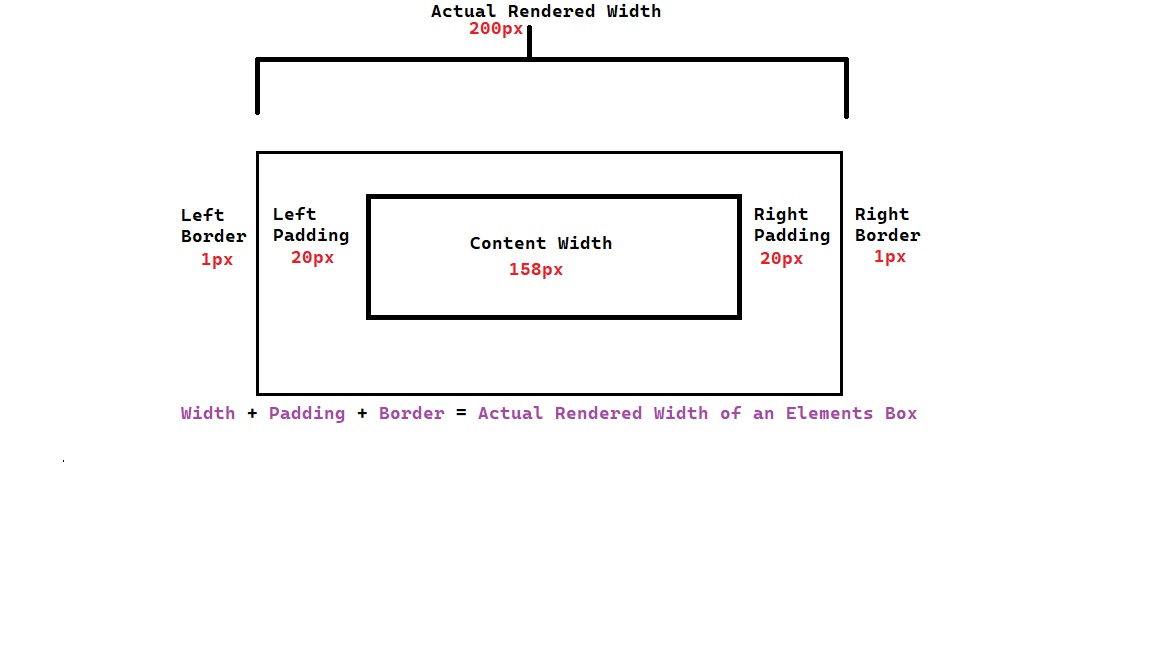
Box-Model:Border-Box
To reset the entire box-model and specify a new one use the following code.
*{
box-sizing: border-box;
}
Margin-Collapse
Margin collapse is simply the combination of the top and bottome margins of blocks, into one single margin with the same size as the largest of these two margins in discussion.
Note: Only occurs with vertical margins. Not horizontally.
Overflow
overflow
is a property which determines solution of an issue in the scenario of content being too large for it's container.
Default value: visible
(content will not be altered and it will just take the space in which it is overflowing.)
Other values include ;
scroll
-overflowing content is accessible through scrolling inside the container element.hidden
-as you can imagine based on the value name, the overflowing content will be hidden.
Visbility
visibility
is a property in which an item may be specified to become hidden
.
Note:Does not remove specified element or item from the page. This is to allow the page to stay in-tact and remain organized and structured.
CSS-Padding
Short-hand Property- Allows specification of all padding properties as values on a single line.
Short-hand Property Order(4 Values)
- Padding-Top(6px)
- Padding-Right(11px)
- Padding-Bottom(4px)
- Padding-Left(9px)
p.content-header {
padding: 6px 11px 4px 9px;
}
Short-hand Property Order(3 Values)
- Padding-Top(5px)
- Padding-Right | Padding-Left(10px)
- Padding-Bottom(20px)
p.content-header {
padding: 5px 10 px 20px;
}
Short-hand Property Order(2 Values)
- Padding-Top | Padding-Bottom(5px)
- Padding-Right | Padding-Left(10px)
p.content-header {
padding: 5px 10px;
}